Blog
Updates from the Arroyo team
How to build a plugin system in Rust
Software used by businesses often needs to be extensible. For Arroyo, a real-time SQL engine, that means supporting user-defined functions (UDFs). But how can we support dynamic, user-written code in a static language like Rust? This post dives deep into the technical details of building a dynamically-linked, FFI-based plugin system in Rust.
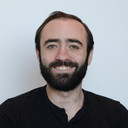
Micah Wylde
CEO of Arroyo
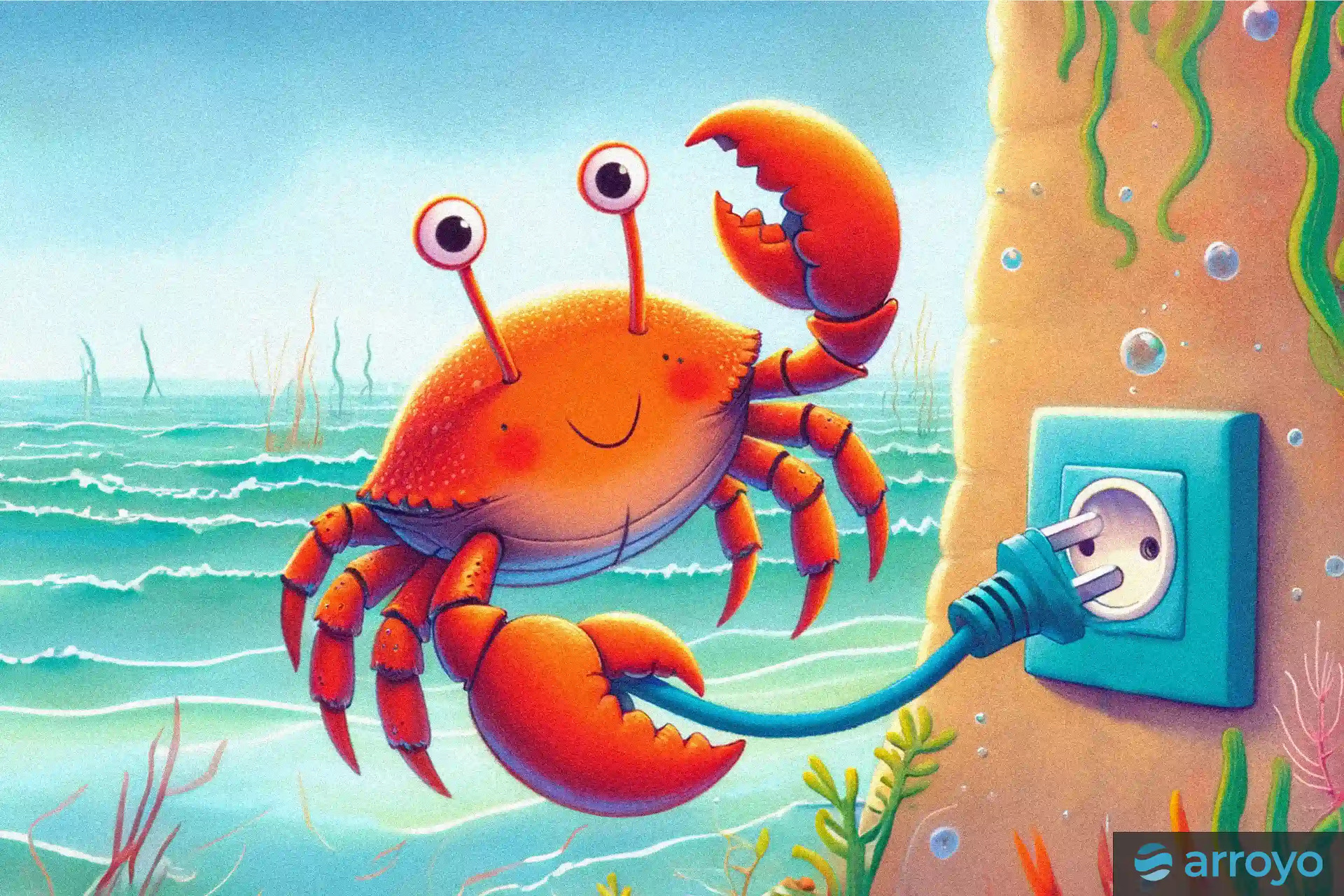